Piezo Buzzer
Arduino Projects ·Introduction
Hey Guys! I hope all of you are doing really great! Today i’ll be showing you how to use a Piezo Buzzer to produce sound.
Note: If you are new to IOT and don’t know anything about programming or Hardware, these Blogs are for you as i’ll be starting from very basic and will be explaining everything. So all you need is an urge to learn!
Modules Used:
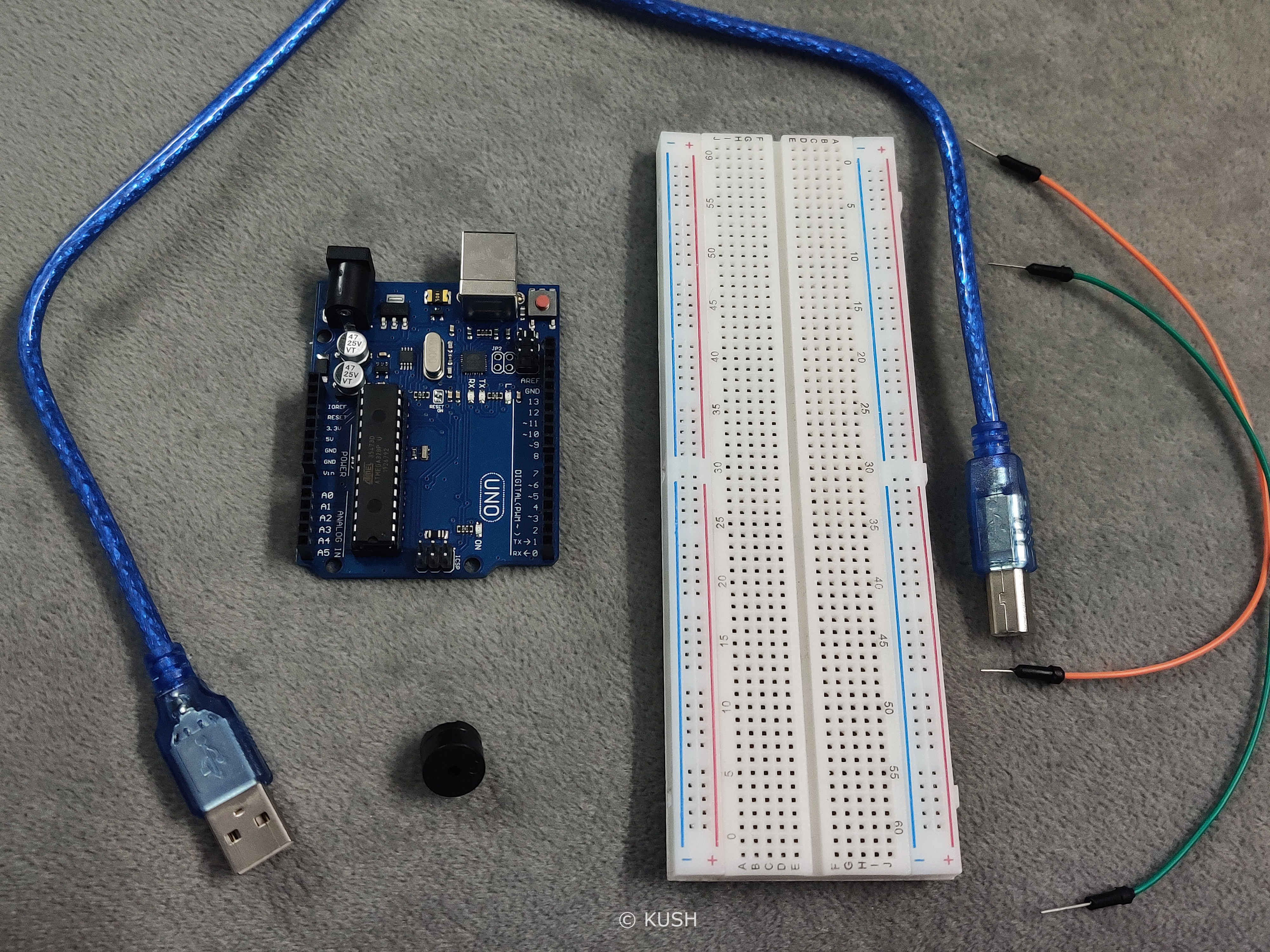
- A Platform for the software End. Laptop, PC etc. with Arduino IDEinstalled.
- An Arduino (Arduino UNO R3 suggested). Note: If you don’t have an Arduino, you can use it virtually
- USB cable to power Arduino
- Two Jumper wires for connection
- Breadboard
- Piezo Buzzer
Got everything? Okay! We’re good to go.
Components Explanation:
Before we start with the assembly of the Hardware, it’s better to understand the use of the components.
Breadboard is a device used for making temporary connections with Arduino. It is used to avoid soldering. Breadboard’s interface is really easy to understand and use. It is divided into three parts. The leftmost and rightmost portions consist of two vertical columns each, with many holes which are connected to each other. That means if we connect one hole of that row to power, the whole vertical row gets connected to power. These holes are labelled as ‘+’ and ‘-‘. Generally the ‘+’ sign row, marked with red is used for providing power to the components and the ‘-‘ sign row, marked with blue is used for grounding the components.
Note: Grounding is connecting the components to Earth in order to prevent overflow of current at the time of accidental cut.
The middle portion consists of horizontal rows with holes in a group of five, which are connected to each other. That means if we connect one hole of that group to certain pin on Arduino, the whole horizontal group of five gets connected to it.
USB wire is used to power the Arduino. We can also use a power adapter but for our project, power supply through USB is enough. Jumper wires are used to connect the components.
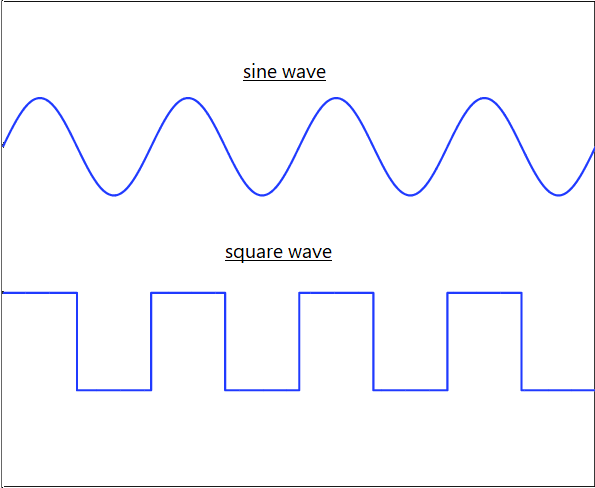
Piezo Buzzer is a device used to generate basic beeps and tones. It generates square waves which are not pleasant to hear with comparison to sine waves due to instantaneous rises and falls.
Hardware Assembly:
Now, let’s start with assembling the Hardware:
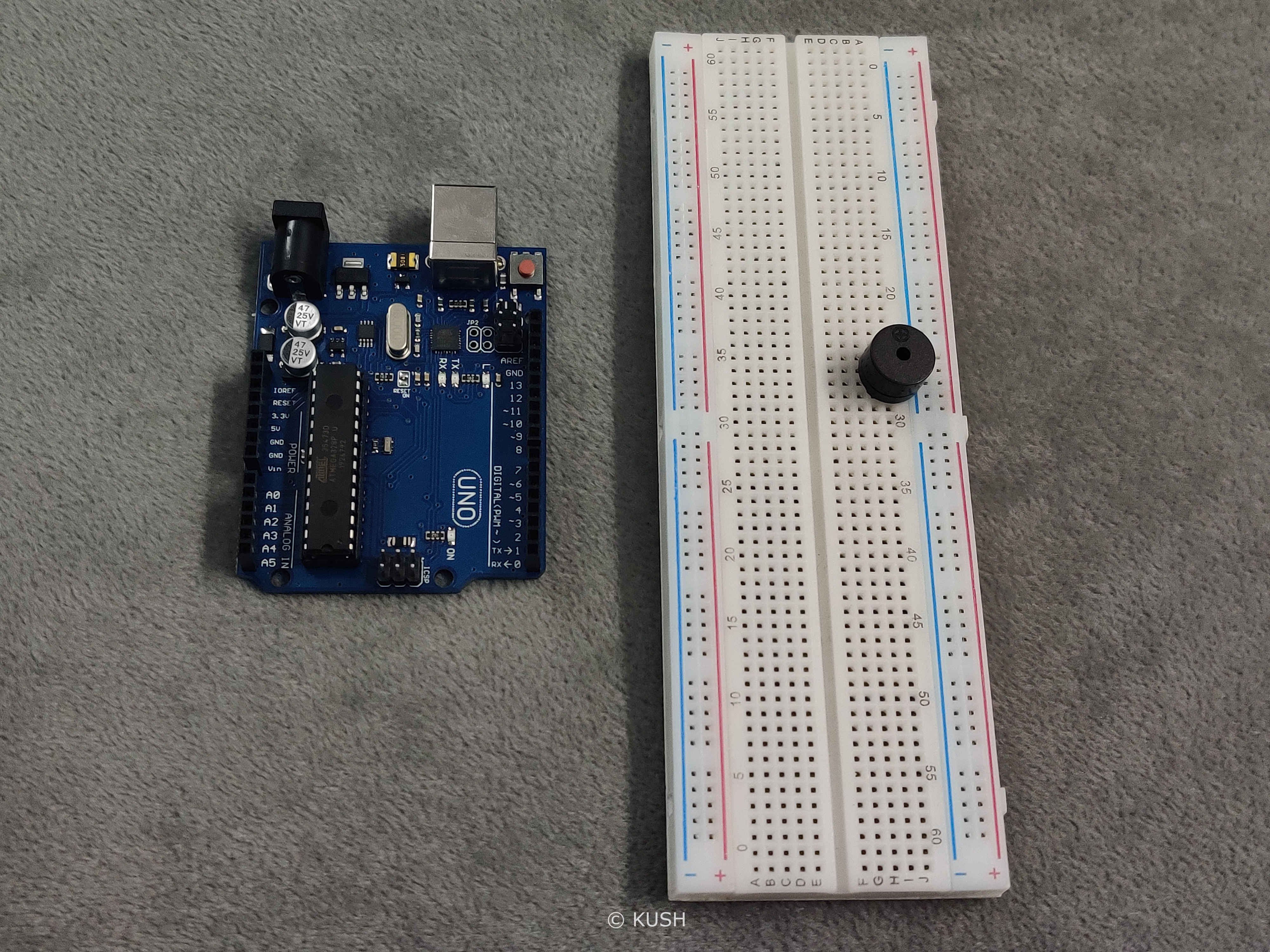
- Firstly connect the Piezo buzzer to Breadboard
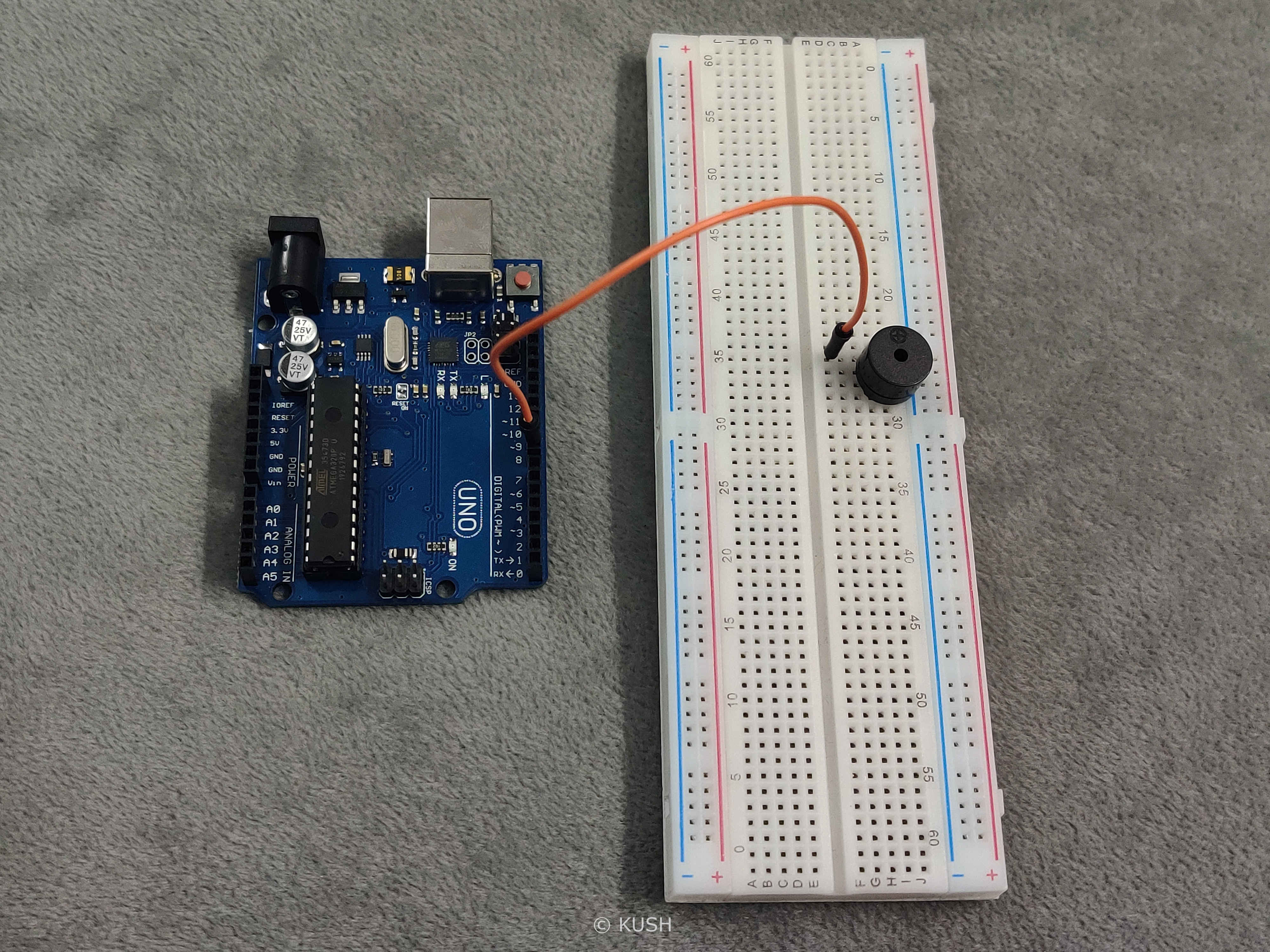
- Now take a jumper wire. Connect it’s one end to the digital Pin8 on the Arduino and other end to one of the leg of Piezo buzzer
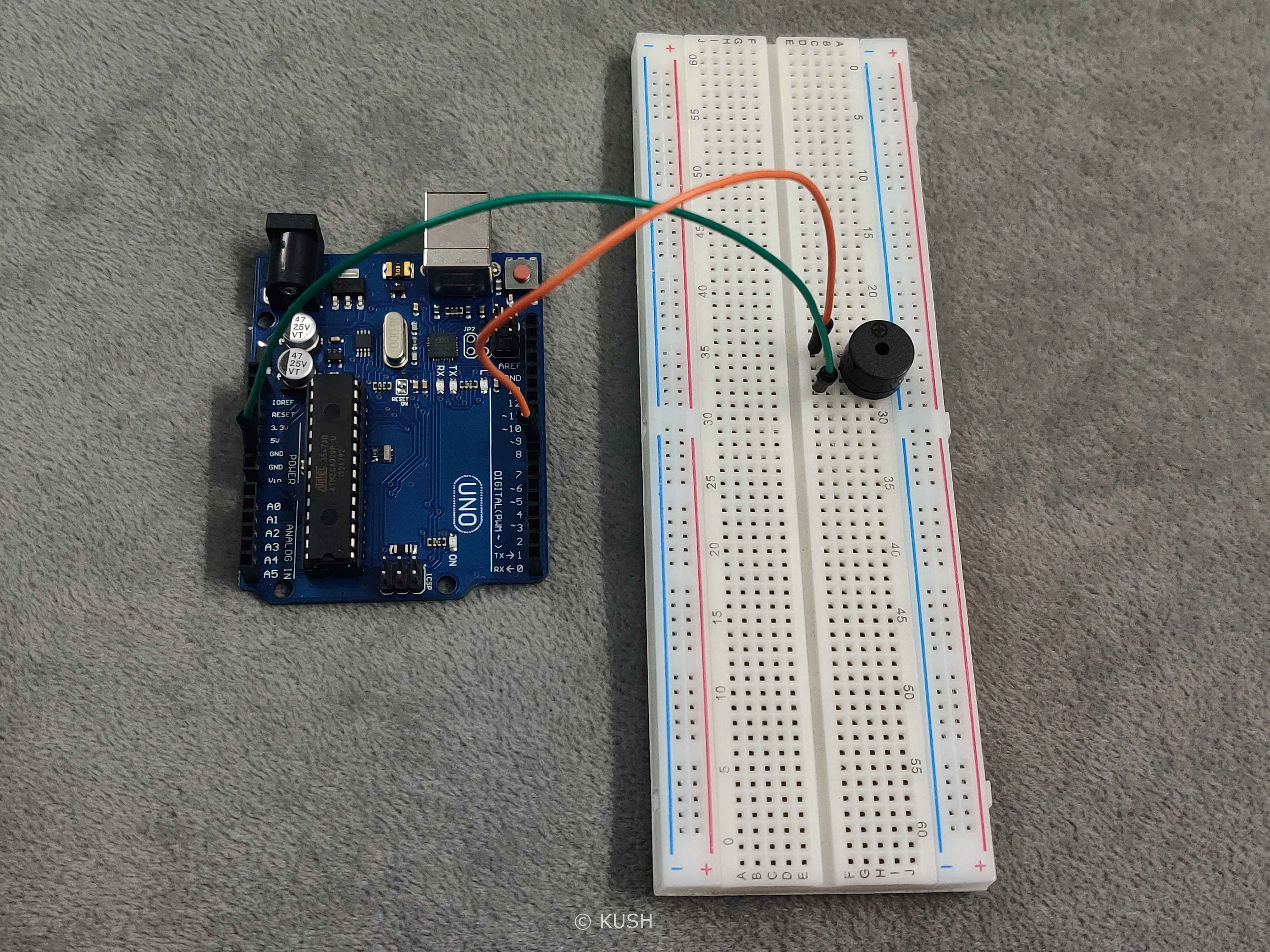
- Lastly take another jumper wire and connect it’s one end to the Ground pin on the Arduino and other end to another leg of Piezo buzzer
Hardware Explanation:
As Jumper wire connected to ground pin on the Arduino and one leg of the Piezo buzzer are in the same group of horizontal holes, therefore they
get connected. Therefore the buzzer is grounded which protects it from excess current.
Also Jumper wire connected to Pin8 on the Arduino and another leg of buzzer are in the same group of horizontal holes, therefore they
get connected. In our case the tone will be produced on pin8.
As the assembly of hardware is done, now it’s time for writing the program and uploading it to the Arduino.
Note: For programming the Arduino, C/C++ language is used. If you don’t know anything about C, just make sure to pay more attention to it. I’ve explained the code and also added comments within the program for better understanding.
Program:
const int buzzer = 8;
void setup(){
}
void loop()
{
tone(buzzer, 1000);
delay(500);
noTone(buzzer);
delay(500)
}
Program Explanantion:
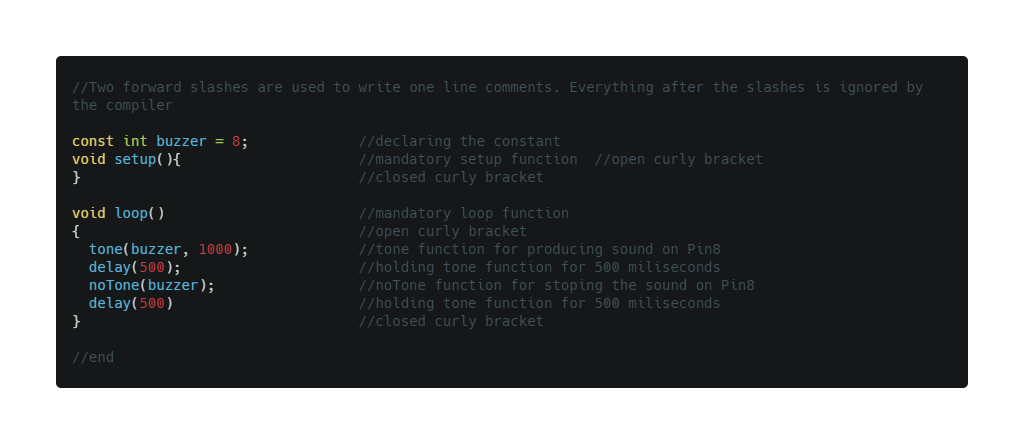
In C language, the first step is to declare the constant or variables that we are going to use in the program. Here, by using ‘const int buzzer = 8;’ we declare that “buzzer” is an integer with a constant value 8, which is our desired pin on the Arduino.
Now, in Arduino sketch the “void setup” and “void loop” are the two main functions which are mandatory for every Arduino
sketch. The ‘Setup’ function is only executed once at the beginning of the program and the ‘loop’ function executes repeatedly.
The term ‘void’ here is used to signify that these functions do not return anything and they don’t take any arguments
(Note: Argument is as a value provided to the function whenever we call it. They are passed inside the bracket ‘()’ by the function).
The curly brackets ‘{}’ after any statment is used to define a clear scope and is also used to group statements which are to be executed
(Note: an open curly bracket must always be closed after grouping the statements)
For this project we don’t need to write anything to Setup. Now in order to make a sound using Piezo buzzer, we will use a function called ‘tone’ which takes two arguments. First is the Pin value for OUTPUT and second is the frequency of the square wave i.e. frequency of the tone. Therefore, the function ‘tone(buzzer, 1000);’ will generate a 1000Hz frquency Square wave or tone at Pin8 and delay(500)’ holds ‘tone’ functon for 500 miliseconds.’ The function ‘noTone’ as the name suggests is used to stop any square wave or tone at the specified pin. Therefore, the function ‘noTone(buzzer);’ stops the sound on Pin8 and ‘delay(500)’ holds ‘noTone’ functon for 500 miliseconds.
You might be wondering that what is the use of a semicolon ‘;’ after every piece of code. Well, in C/C++ semicolon acts as a Terminator and it used to tell the compiler that we have reached the end of the command. Semicolon at the end of a command is mandatory in C/C++ language.
Now we are done with our Program and Hardware. It’s time to upload the sketch to the Arduino.
- Use the USB cable provided with Arduino to connect it with the PC.
- Use ‘Upload’ to verify and upload the sketch to the Arduino.
Note: If you get any errors while compiling the sketch, just make sure the Arduino is connected correctly to the PC and the ‘board’ & ‘port’ is specified in the tools menu. Also check for any errors in the code itself, especially look for unclosed curly brackets or missing semicolons at the end of the statements.
Once the program is successfully verified and uploaded to the Arduino we will see a ‘Done Uploading’ message at the bottom of the screen and the PiezoBuzzer starts buzzing.
You can also modify the code for different sound patterns.
For Example:
const int buzzer = 8;
int i;
int j;
void setup() {
}
void loop() {
for(i=0; i<6; i++){
tone(buzzer, 1000);
delay(100);
noTone(buzzer);
delay(100);
tone(buzzer, 2000);
}
for(j=0; j<2; j++){
tone(buzzer, 2000);
delay(300);
noTone(buzzer);
delay(300);
}
}
Conclusion:
In this blog we learnt how to produce a tone using a Piezo Buzzer.
This was just a basic Example; We will keep stepping up. So for many more such projects, stay tuned!
Thank You!
Share on: